mirror of https://github.com/pret/pokeemerald.git
Starting the copying process for basic scripting tutorial
parent
1aea433cf3
commit
246f86b762
|
@ -0,0 +1,125 @@
|
|||
Originally published by [Avara](https://www.pokecommunity.com/member.php?u=294199) on [PokeCommunity](https://www.pokecommunity.com/showthread.php?t=416800).
|
||||
|
||||
-----
|
||||
|
||||
If you already know XSE scripting from binary hacking, you should be able to pick up scripting in the decomps without too much trouble. However, if you're totally new to ROM hacking and want to begin with Pokéemerald, this guide will hopefully be of some use to you!
|
||||
|
||||
To start, you don't need any special scripting tools such as XSE - all you need is a decent text editor (such as Notepad++ or VSCode) and [PoryMap](https://github.com/huderlem/porymap/releases) to get started! It's easy to modify existing scripts - you simply edit the “scripts.inc” file as needed and hit "Save".
|
||||
|
||||
|
||||
# Labelling
|
||||
|
||||
If you look in “data\maps”, you'll find folders pertaining to each in-game map. Each of these map folders has their own “scripts.inc” file, where the scripts for each map are contained. You can also open a map's scripts by clicking "Open Map Scripts" in the top right corner of PoryMap's Events tab.
|
||||
|
||||
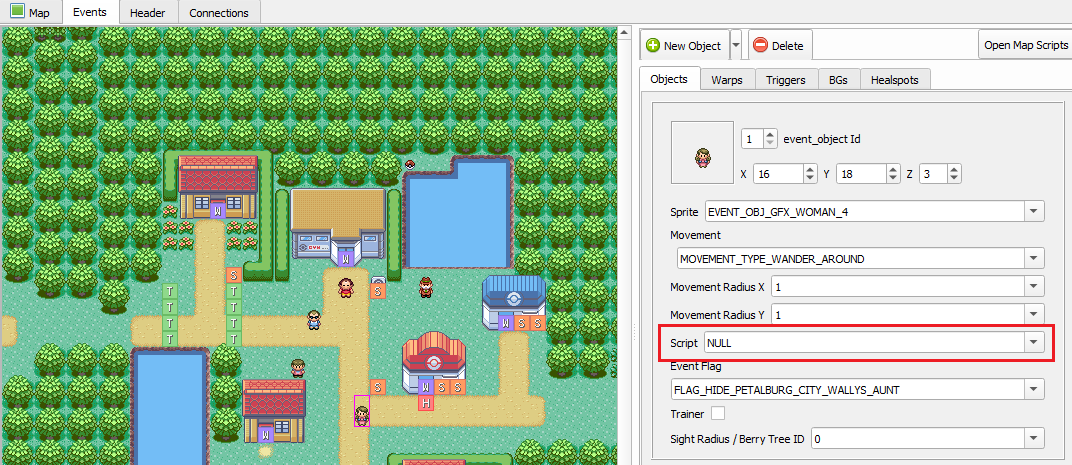
|
||||
|
||||
You can technically name your labels whatever you like so long as they are unique, but it's recommended that you stick to some kind of pattern when you're naming them so you don't confuse yourself. In this guide, I'll be using `Script_Something` for script labels and `Text_Something` for text labels for simplicity's sake, but if you have a look at existing scripts, you'll notice that a lot of labels are along the lines of `MapName_Something`/`MapName_SomethingText`.
|
||||
|
||||
To assign a script to a map object, you'll want to highlight it and put the script's label in the "Script" box outlined in red above.
|
||||
|
||||
|
||||
# Simple Text Scripts
|
||||
|
||||
Here is an example of a simple text script:
|
||||
|
||||
<img align="right" src="https://user-images.githubusercontent.com/794812/222920483-f217de5a-0262-4e61-9aae-000bbf92a048.gif">
|
||||
|
||||
```gas
|
||||
Script_SimpleMsgScript::
|
||||
lock
|
||||
faceplayer
|
||||
msgbox Text_SimpleMsgScript, MSGBOX_DEFAULT
|
||||
release
|
||||
end
|
||||
|
||||
Text_SimpleMsgScript:
|
||||
.string "Hi!\n"
|
||||
.string "My name is Adam.\l"
|
||||
.string "What's your name?\p"
|
||||
.string "Nice to meet you, {PLAYER}!$"
|
||||
```
|
||||
|
||||
Let's break down the script:
|
||||
|
||||
- `lock` prevents the player from moving.
|
||||
- Alternatively, `lockall` stops all NPCs on the map from moving.
|
||||
- `faceplayer` makes the NPC or object the player just talked to face the player.
|
||||
- `msgbox` we'll get into below.
|
||||
- `release` lets the player move again.
|
||||
- If you used `lockall` instead, you'll have to use `releaseall`.
|
||||
- `end` indicates the end of the script (obviously).
|
||||
|
||||
For the text part:
|
||||
- `.string` indicates a line of in-game text.
|
||||
- `{PLAYER}` is a "placeholder" that displays the player's name.
|
||||
- `\n` moves text printing to the next line.
|
||||
- `\l` is only used after the initial use of `\n` to scroll down to the next line after the player presses a button.
|
||||
- `\p` also waits for play input then clears the display before continuing. Good for new sentences.
|
||||
- `$` indicates the end of the text. Make sure this is at the end of your text, otherwise the game will continue to print garbage afterwards.
|
||||
|
||||
The `msgbox` line contains our label for the text to be displayed, as well as the type of msgbox.
|
||||
- `MSGBOX_DEFAULT` - If you don't supply a type, it defaults to this type. This type will display the text and then wait for the player to press any button before continuing the script.
|
||||
- `MSGBOX_NPC` - This type of msgbox does `lock`, `faceplayer`, and `release` for you. Used most often with NPCs.
|
||||
- `MSGBOX_SIGN` - This type of msgbox does `lock` and `release` for you. Used most often with signs.
|
||||
- `MSGBOX_YESNO` - This type of msgbox presents a Yes/No option once it's finished printing. (See also: the `yesnobox` command.)
|
||||
|
||||
Here's an example of MSGBOX_NPC:
|
||||
|
||||
```gas
|
||||
Script_SimpleNPCScript::
|
||||
msgbox Text_SimpleNPCScript, MSGBOX_NPC
|
||||
end
|
||||
|
||||
Text_SimpleNPCScript:
|
||||
.string "Hi!\n"
|
||||
.string "My name is Adam.$"
|
||||
```
|
||||
|
||||
Here's an example of MSGBOX_SIGN:
|
||||
|
||||
```gas
|
||||
Script_SignpostScript::
|
||||
msgbox Text_SignpostScript, MSGBOX_SIGN
|
||||
end
|
||||
|
||||
Text_SignpostScript:
|
||||
.string "Pokémon Herb Shop\n"
|
||||
.string "Bitter taste, better cure!$"
|
||||
```
|
||||
|
||||
Here's an example for MSGBOX_YESNO:
|
||||
|
||||
```gas
|
||||
Script_SimpleYesNoScript::
|
||||
lock
|
||||
faceplayer
|
||||
msgbox Text_SimpleYesNoScriptQuestion, MSGBOX_YESNO
|
||||
compare VAR_RESULT, 0
|
||||
goto_if_eq Script_PlayerAnsweredNo
|
||||
msgbox Text_PlayerAnsweredYes, MSGBOX_DEFAULT
|
||||
release
|
||||
end
|
||||
|
||||
Script_PlayerAnsweredNo::
|
||||
msgbox Text_PlayerAnsweredNo, MSGBOX_DEFAULT
|
||||
release
|
||||
end
|
||||
|
||||
Text_SimpleYesNoScriptQuestion:
|
||||
.string "Dragon-type Pokémon are the best!\n"
|
||||
.string "Wouldn't you agree?$"
|
||||
|
||||
Text_PlayerAnsweredYes:
|
||||
.string "Yeah! Dragons rule!$"
|
||||
|
||||
Text_PlayerAnsweredNo:
|
||||
.string "I guess you've never raised one.$"
|
||||
```
|
||||
|
||||
A few more notes about MSGBOX_YESNO:
|
||||
- The `compare VAR_RESULT` line checks the result of the player's answer - 0 for "No", 1 for "Yes". More about vars later!
|
||||
- The `goto_if_eq` line means the script will go to a new label if the result is equal to what we specified above, in this case, 0. If it's *not* equal to that, the script will continue as normal.
|
||||
|
||||
|
||||
|
||||
|
Loading…
Reference in New Issue